Device Management Agent : Java Agent Documentation¶
DM agent is a LWM2M client. We have two versions of DM agent, one is Java based client and the other is C based client.
Java Agent Overview¶
Device Management is based on OMA LightweightM2M standard. Device agent is LWM2M client instance and DM server is LWM2M server instance. LWM2M core is provided by DMClient SDK Library (DCSDK) based on Leshan.
Every device parameter can be conceived as a resource. Each resource is a COAP restful resource which can be created, read, updated, deleted or executed.
An Object is a collection of resources of similar nature.
A Client has one or more Object Instances. Objects/Resources are accessed with restful URIs through CRUD methods.
Object Instances have an associated Access Control Object Instance containing Access Control Lists(ACLs) that define which logical operations (CRUDE) are permissible.
Agent Architecture¶
The DM agent architecture is depicted thorough the following image:
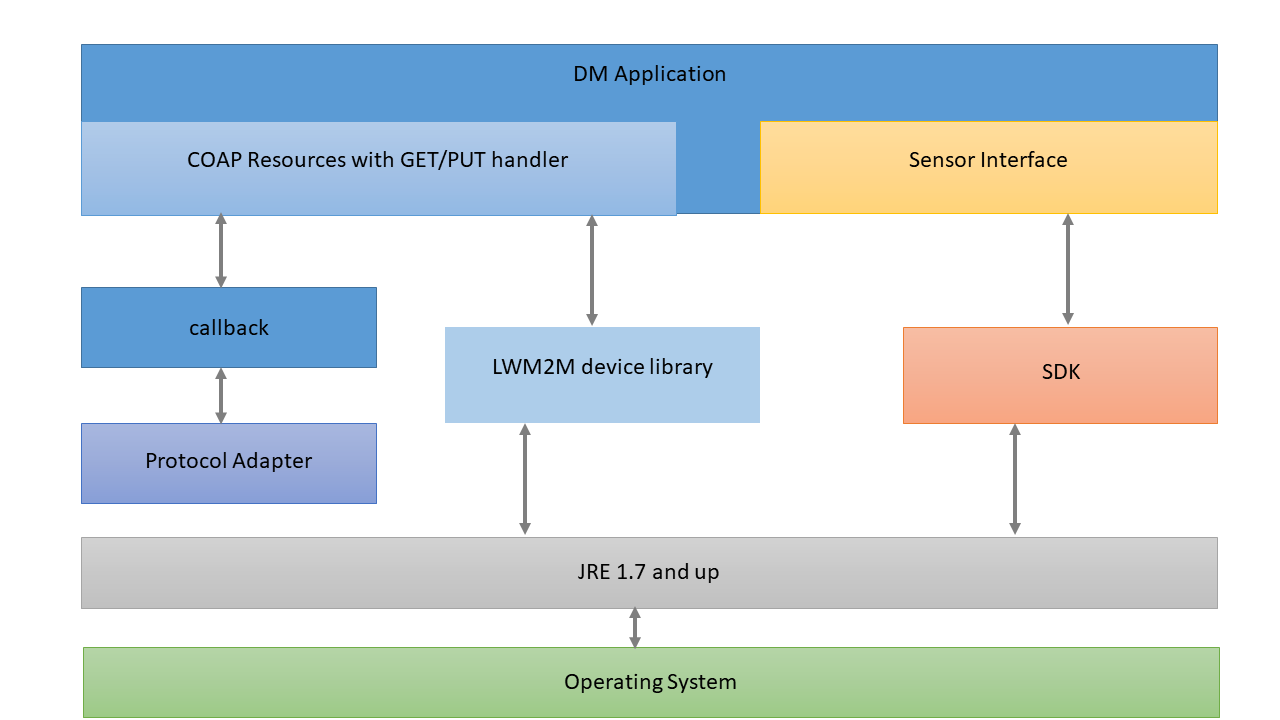
Pre-requisite¶
Add dmclient-sdk.jar to build path
Dependency : commons-cli-1.2.jar
Import the following packages:
commons-cli-1.2.jar library includes these :
import org.apache.commons.cli.CommandLine; | import org.apache.commons.cli.CommandLineParser; | import org.apache.commons.cli.HelpFormatter; | import org.apache.commons.cli.OptionBuilder; | import org.apache.commons.cli.Options; | import org.apache.commons.cli.ParseException; | import org.apache.commons.cli.PosixParser;
dmclient-sdk.jar library includes these :
import org.eclipse.californium.scandium.ScandiumLogger;import org.eclipse.leshan.dms.clientsdk.constants.UrlMapper;import org.eclipse.leshan.dms.clientsdk.core.BasicCoapResource;import org.eclipse.leshan.dms.clientsdk.core.Command;import org.eclipse.leshan.dms.clientsdk.core.DeviceRegister;import org.eclipse.leshan.dms.clientsdk.core.DeviceRegister.State;import org.eclipse.leshan.dms.clientsdk.core.TcoapServer;import org.eclipse.leshan.dms.clientsdk.core.bootstrap.BootstrapResponse;import org.eclipse.leshan.dms.clientsdk.core.bootstrap.ConfigSettings;import org.eclipse.leshan.dms.clientsdk.core.bootstrap.EndpointBootstrap;import org.eclipse.leshan.dms.clientsdk.core.bootstrap.EndpointBootstrap.BootstrapState;import org.eclipse.leshan.dms.clientsdk.core.security.Lwm2mSecurity;import org.eclipse.leshan.dms.clientsdk.core.security.SecurityIdentity;import org.eclipse.leshan.dms.clientsdk.exception.DecryptionException;import org.eclipse.leshan.dms.clientsdk.exception.SecurityKeyException;
Basic Steps in a Java Agent¶
Let us go through the steps with the sample codes. In the given java sample lwm2mclient.java contains the resource handlers. Example project workspace can be imported as java project in Eclipse.
Define DM server¶
Define URI, device port and DM user key who owns the device:
Sample Code
InetSocketAddress inet = new InetSocketAddress(leshanip, serverPort);
TcoapServer server = new TcoapServer();
server.setLifeTime(3600L);
server.create(clientPort,inet);
server.enableHeartBeat(true);
Enable DTLS PSK and identity:
Sample Code
if(identity!=null && psk!=null){
Lwm2mSecurity sec = server.setEnableDTLS(true);
sec.setLwm2mServerURI("coap://127.0.0.1:5684");
sec.setBootstrapServer(false);
sec.setSecurityMode(Lwm2mSecurity.mode.PSK);
sec.setSecurityInfo(SecurityIdentity.newPreSharedKeyInfo(identity, psk.getBytes()));
}
Add Device Resources¶
Each device parameter is added as COAP resource. There are two types of resources: * Static resource - value constant like make, model etc. * Dynamic resource - value changing like CPU load, free memory etc.
Sensor resource is also dynamic resource like light, accelerometer etc. Needs callback functions invoking sensor API
A COAP restful resource is defined in two steps:
Define COAP resource for a static resource
Let us define a static resource “MAC”
Define COAP resource URI
For MAC we can define COAP URI as | server.addAndMapResources(UrlMapper.MAC_ID, macid);
Now Let us define a dynamic resource “System Up Time” | server.addAndMapResources(“/15/0/5”, new SysUpTime());
Note: LWM2M allows resource URL in object/object instance/resource format. Though there is no mandatory resource URL but the standard prescribes certain resources called normative resources as per specific URL. Normative resources like MAC, IMEI are clubbed together under UrlMapper class.
In TCUP all sensor resources are put under object 15 for example a freeRAM under /15/0/6 etc. Custom resources are advised to be put under object 17 for e.g. a restart command under /17/0/16 etc.
The resource values should only have alpha numeric characters.
Add callback function with Get Put handlers for COAP resource class for dynamic resource.
The SystemUpTimeResource class will contain the GET PUT handlers with callback to acquire real value. Example code
public class SysUpTime extends AbstractsubscribableResource {
private long started;
public SysUpTime() {
this.getLinkHandle().setTitle("systemUpTime");
this.getLinkHandle().setObservable(true);
this.getLinkHandle().setParameters("ut", "seconds");
this.started = System.currentTimeMillis();
ScheduledExecutorService ses = Executors.newScheduledThreadPool(1);
ses.scheduleWithFixedDelay(new Runnable() {
public void run() {
notifyChange();}
}, 10, 5, TimeUnit.SECONDS);}
@Override
public void handleGET(CoapExchange ex) {
String time = getSysUpTime(); // function to get system up time
System.out.println("system up time is :"+time);
ex.respond(ResponseCode.CONTENT, time);}
iv) Set Attribute of resource: In constructor set following attributes
this.getLinkHandle().setTitle("systemUpTime");
this.getLinkHandle().setObservable(true);
setObservable(Boolean.TRUE) //resource can be subscribed for automatic notification
Set Additional Attribute:
For setting up unit use “ut”
For setting up datatype use “dt”
For setting it editable use “upt”
v)Likewise other static or dynamic resources are defined.
Registration¶
Once the resources are defined, register the device with DM server.
Sample CODE
DeviceRegister register = new DeviceRegister (server,endpointName);
register.register(new Command<EndPointRegistrator.RegistrationState>() {
public void callException(Exception e) {
System.out.println(e); }
public void call(RegistrationState t) {
System.out.println("registration state :"+t);}
Registration state will return the following status to agent:
200 for successful registration.
403 for registration denial for device name already taken.
Steps to Run:¶
Preregister a device using the portal or API sandbox.
Generate PSK for the preregistered device using portal or API sandbox, DTLS identity and PSK will be generated.
Register the device by running device agent with generated PSK and identity argument.
Example Run:
java -jar dmagent.jar -ip “10.100.10.96” -key “key#demo” -ep “dtlsleshan” -id “PADjdBcdGpf3vKqC” -psk “BG4pDrcZ0w2W4Dv4” -p 5684