Execute and View Result¶
Input data can be posted to MR through the following three mediums:
SOS : SOS is Sensor Observation Service which registers the sensors and posts observation JSON to MR.
DM : DM is Device Management service which registers the devices which posts data directly to MR.
SendRequest API: This is an additional API available in MR for posting data to MR input. Below is the detailed operation of send request API.
Publish Observation Data¶
SendRequest(HTTP)¶
sendRequest() API allows user to post messages to direct/topic types of exchanges or a queue using REST API.
The header of the web service must set to content type application/JSON along with DirectExchangeName and RoutingKey.
Custom Request Headers for send request
x-api-key : a mandatory header to pass along with every API call.
x-app-key : an optional header to pass APP key for the calling application.
type : a query parameter to define type of exchange or queue, default is direct type of exchange. topic and queue are the two other options.
DirectExchangeName : Direct Exchange name as mentioned in the routing rule. Default is Direct.Exchange used by SOS. In case if type is topic/queue, then put topic/queue name here.
RoutingKey : This should be same as mentioned in the routing rule. Default is tenant unique key used by SOS. If it is blank default unique value for the tenant will be populated.
ContentType : This should be set as application/JSON.
JSON for Single Send Request
sendRequest() API allows user to send any valid JSON , but in order to process it by MR rule, it should follow SOS JSON format.
{
"version": "1.0.1",
"observations": [
{
"sensor": "NEXUS1",
"feature": "room1",
"record": [
{
"starttime": "1-JAN-2014 15:30:01 IST",
"output": [
{
"name": "OutsideSensorTemp",
"value": "46.0",
"type": "decimal"
},
{
"name": "temp",
"value": "99",
"type": "quantity"
}
]
}
]
}
]
}
Following is the URL for MR send request:
POST http://<domain>/MessageRouting/v2.0/sendRequest
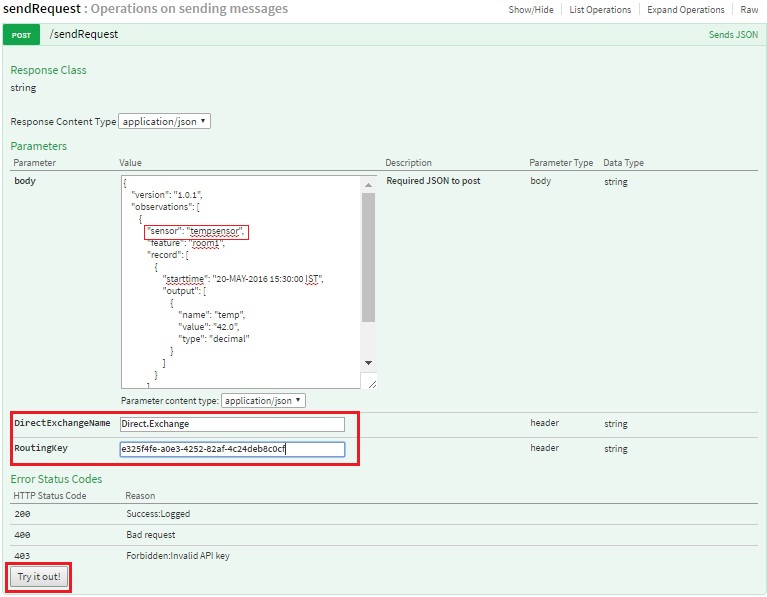
JSON for Bulk Send Request
sendRequest() API allows user to send any valid JSON , Internally it streams the JSON array.
[
{
"version": "1.0.1",
"observations": [
{
"sensor": "NEXUS1",
"feature": "room1",
"record": [
{
"starttime": "22-AUG-2019 15:30:01 IST",
"output": [
{
"name": "temp",
"value": "99",
"type": "quantity"
}
]
}
]
}
]
},
{
"version": "1.0.1",
"observations": [
{
"sensor": "NEXUS1",
"feature": "room1",
"record": [
{
"starttime": "22-AUG-2019 15:40:01 IST",
"output": [
{
"name": "temp",
"value": "100",
"type": "quantity"
}
]
}
]
}
]
}
]
Following is the URL for MR send bulk SOS JSON :
POST http://<domain>/MessageRouting/v2.0/sendRequest/bulk
sendRequest() API can also send bulk SOS formatted JSON by sending array of JSON document. Internally it streams the JSON array.
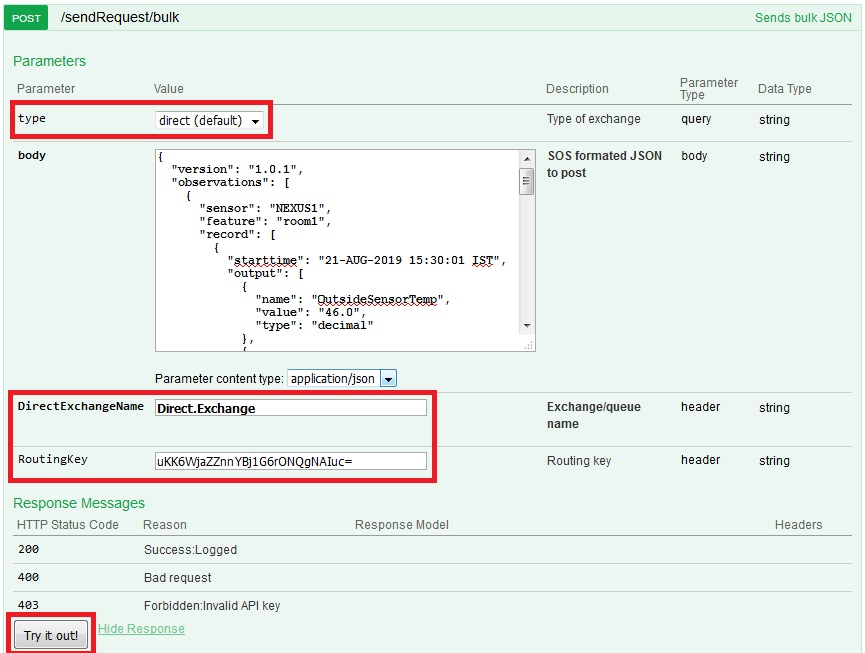
{
"version": "1.0.1",
"observations": [
{
"sensor": "NEXUS1",
"feature": "room1",
"record": [
{
"starttime": "1-JAN-2014 15:30:01 IST",
"output": [
{
"name": "temp",
"value": "99",
"type": "quantity"
}
]
}
]
},
{
"sensor": "NEXUS1",
"feature": "room1",
"record": [
{
"starttime": "1-JAN-2014 15:30:01 IST",
"output": [
{
"name": "temp",
"value": "100",
"type": "quantity"
}
]
}
]
}
]
}
Note
sendRequest() API can post data to direct exchange/topic exchange or queue
Once user clicks Try it out!
button the data is sent and a success message is shown as response from API swagger.
SendRequest(gRPC)¶
The gRPC compliant version of the sendRequest API.This will allow users to post messages to the Exchange using gRPC protocol. It allow to users to invoke 3 types of API :
1. Send any valid json
2. Send SOS schema based JSON
3. Send any binary payload
User can build client gRPC stub using any language and invoke above API to send data to the gRPC server. The gRPC server will receive the data and post it to the exchange as mentioned in the header parameters.
The protocol buffer specification :
syntax="proto3";
option java_multiple_files=true;
option java_package="messageroutersos";
option java_outer_classname="MessageRouterProtoSos";
option objc_class_prefix = "HLW";
message Request { //for posting any format json
string data =1;
}
message Reply {
string response = 1;
}
message SosRequest{ //for posting SOS format json
string version = 1;
message OBSERVATIONS {
string sensor = 1;
string feature = 2;
string offering=3;
string validUpto=4;
string privacy=5;
message RECORD {
string starttime = 1;
string endtime=2;
string associatedObservation=3;
message POSITION_GLOBAL {
string latitude = 1;
string longitude = 2;
string altitude = 3;
}
POSITION_GLOBAL position_global = 4;
message POSITION_LOCAL {
string name = 1;
string value = 2;
string type = 3;
string unit = 4;
string crs = 5;
}
repeated POSITION_LOCAL position_local = 5;
message OUTPUT {
string name = 1;
string value = 2;
string type = 3;
}
repeated OUTPUT output = 6;
}
repeated RECORD record = 6;
message PARAMETER {
string name = 1;
string value = 2;
string type = 3;
string unit = 4;
}
repeated PARAMETER parameter = 7;
message QUALITY {
string name = 1;
string value = 2;
string type = 3;
string unit = 4;
}
repeated QUALITY quality = 8;
message META_DATA {
string name = 1;
string value = 2;
string type = 3;
string unit = 4;
}
repeated META_DATA meta_data = 9;
}
repeated OBSERVATIONS observations = 2;
}
message SosResponse{
string result=1;
}
message BinaryRequest{ //for posting binary data
bytes anybinary=1;
}
message BinaryResponse {
string response=1;
}
service SendRequest { //service for posting any format json
rpc sendRequest(Request) returns (Reply) {}
}
service SendRequestSOS { //service for posting SOS format json
rpc sendRequest(SosRequest) returns (SosResponse) {}
}
service SendRequestBinary { //service for posting binary data
rpc sendRequest(BinaryRequest) returns (BinaryResponse) {}
}
The header will contain the API key along with the DirectExchangeName.
Custom Request Headers for send request
x-api-key : a mandatory header to pass along with every API call. This will be sent from the grpc client end.
DirectExchangeName : The exchange name to where the data will be posted in the message broker. This will be sent from the grpc client end.
Send any valid json
This API allows user to send any valid json data to the gRPC server. The server will receive the json data and post to the mentioned exchange. The json data will be posted to the mentioned exchange along with the content type application/json.
{
"id": "1562237411930-0",
"startTime": "23-JUL-2013 15:30:00.640 IST",
"endtime": "23-JUL-2013 15:30:00.640 IST",
"feature": "bus-1",
"sensor": "sensor1",
"offering": "bus-tracking",
"validUpto": "23-JUL-2023 15:30:00 IST",
"privacy": "public",
"position-local_floor": "4A",
"position-local_floor_type": "text",
"speed": "40.0",
"speed_type": "decimal",
"meta-data_speedometer-version": "3.0",
"parameter_traffic": "heavy",
"parameter_traffic_type": "text",
"quality_accuracy": "99",
"quality_accuracy_type": "number"
}
Send SOS schema based JSON
This API allows user to send any SOS json format data to gRPC server. The server will receive the sos json data and post to the mentioned exchange. The json data will be posted to the mentioned exchange along with the content type application/json.
{
"version": "1.0.1",
"observations": [
{
"sensor": "sensor1",
"feature": "room1",
"record": [
{
"starttime": "1-JAN-2014 15:30:01 IST",
"output": [
{
"name": "OutsideSensorTemp",
"value": "46.0",
"type": "decimal"
},
{
"name": "temp",
"value": "99",
"type": "quantity"
}
]
}
]
}
]
}
Send any binary payload
This API allows user to send any binary data to the gRPC server, like an image,etc. The server will receive the binary payload and post to the mentioned exchange. The binary data will be posted to the mentioned exchange along with the content type application/x-binary.
View Result¶
In order to view result data, the following actions have to be taken:
Create MR Rule : Create MR rule and start the rule. Refer section Create Routing Rule to create a new MR rule.
SSE Viewer : SSE viewer page is used to subscribe the output of MR rule on a particular Topic Exchange.
Invoke sendRequest() API : Send the sensor observation JSON through sendRequest() API.
View Result using SSE Viewer
Server sent event (SSE) is used to subscribe MR rule output, so that we can receive the output from MR rule. The following image shows how to configure the SSE viwer page:
Go to home page of TCUP portal and click on SSE Viewer
service under Services
tab on the left hand side of the portal.
Once the SSE viewer page is launched, user has to provide the details like User API Key , Input Type , Topic Exchange Name and Topic Name if input type is selected as topic and Message Queue Name if input type selected as queue. After providing these details click on Start
button to subscribe the channel as shown in the image below:
Note
Topic Exchange Name and Topic Name should be same as provided in MR rule and rule should be in running status. Before using send request API, user needs to conimage SSE viewer page to view result.
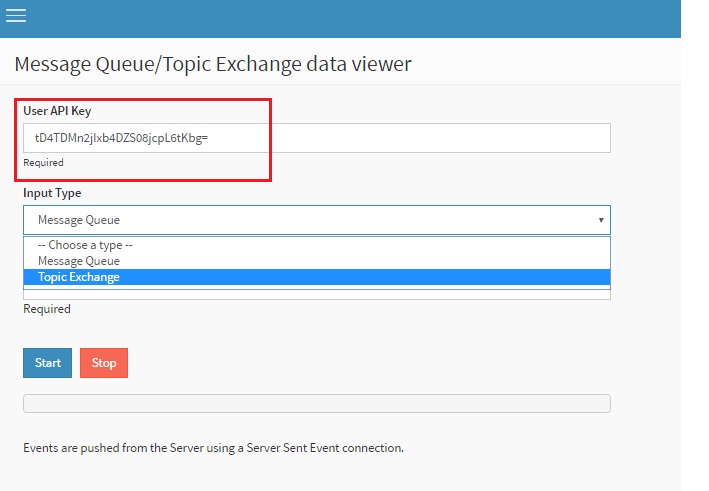
After selecting type as topic, provide Topic Exchange name and Topic name detail and click on
Start
button.
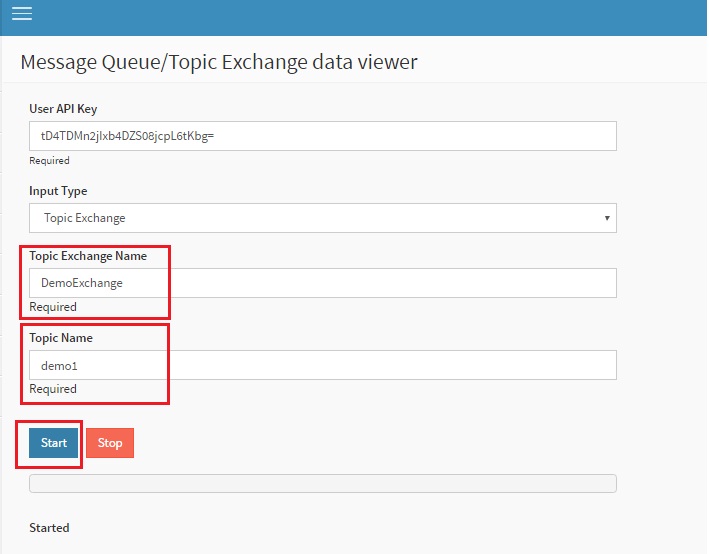
Invoke sendRequest() API
Rules will get the data only if rule input details (exchange name and topic or queue name) matches. Here in our MR rule direct exchange name is Direct.Exchange and Routing Key is e325f4fe-a0e3-4252-82af-4c24deb8c0cf. The same we have provided in our sendRequest() API as shown in the image below:
Input SOS JSON
MR rules can only be successfully executed if observation data follow SOS JSON format, but for MR buffer rule(no filtering condition) any valid input JSON can be executed.
{
"version": "1.0.1",
"observations": [
{
"sensor": "NEXUS1",
"feature": "room1",
"record": [
{
"starttime": "1-JAN-2014 15:30:03 IST",
"output": [
{
"name": "OutsideSensorTemp",
"value": "46.0",
"type": "decimal"
},
{
"name": "temp",
"value": "99",
"type": "quantity"
}
]
}
],
"meta-data": []
}
]
}
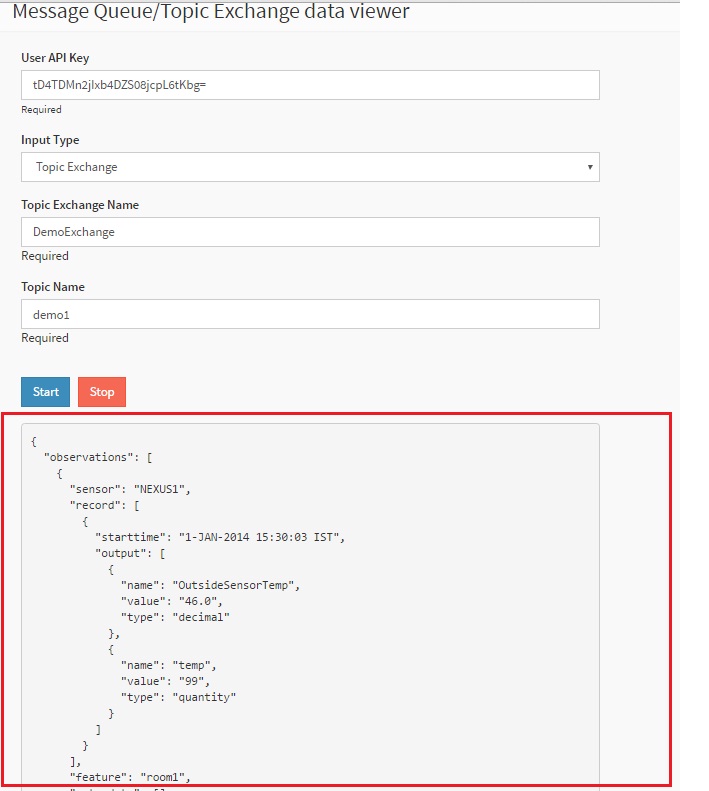
Note
Sensor name of the input JSON data should be same as sensor ID provided in MR rule else the data will be discarded, similarly there are other fields provided in MR rule which acts as filtering condition in MR rule which will be discussed in details later.